Java is a worldwide programming language known for its versatility and robustness. It is utilized in various domains, including web development, enterprise software, Android app development, and more. Developing Java projects involves writing code that performs specific tasks and solves problems.
However, the quality of the code plays a crucial role in determining the effectiveness, maintainability, and longevity of the projects. In this article, we will explore the significance of code quality in Java projects, discuss tips for improving code quality, and introduce useful tools to enhance the overall quality of Java code.
Introduction to Java Projects with Source Code
Java projects are software applications or systems developed using the Java programming language. These projects encompass a wide range of applications, from small scripts to large-scale enterprise systems. Java provides a comprehensive set of libraries, frameworks, and tools that enable developers to build robust and scalable software solutions.
Note: If you want to learn Java Projects with Source Code, click on the given link
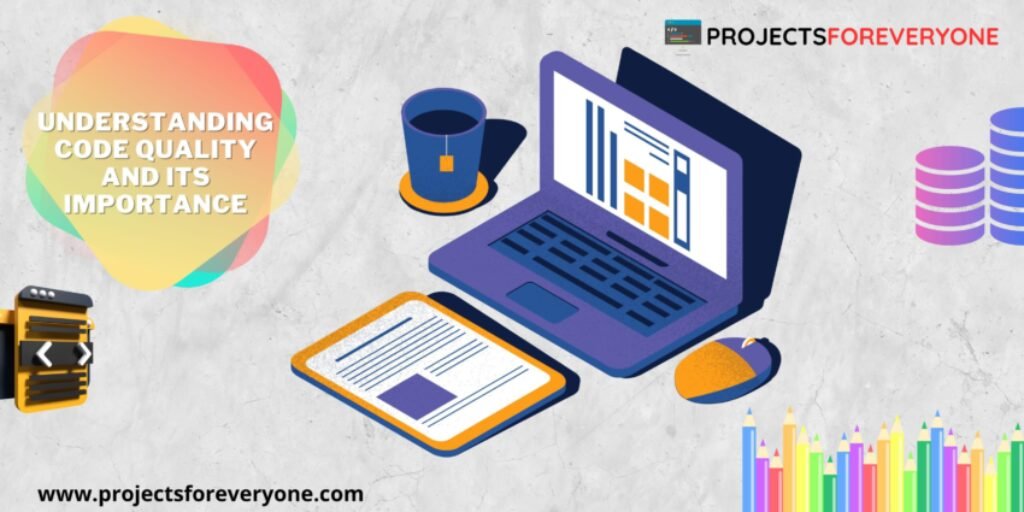
Understanding Code Quality and its Importance
Code quality refers to the measure of how well the code meets certain standards and characteristics, including readability, maintainability, efficiency, and reliability. High code quality is essential as it directly impacts the overall success of a software project. Here are some reasons why code quality matters:
- Readability: Well-written code is easy to understand and navigate, allowing developers to maintain, debug, and extend it efficiently. It promotes collaboration among team members and facilitates knowledge sharing.
- Maintainability: A code that follows best practices and design principles is easier to maintain. As software projects evolve, code needs to be modified, fixed, and enhanced. Good code quality reduces the time and effort required for maintenance tasks.
- Efficiency: High-quality code performs optimally, utilizing system resources effectively. It minimizes unnecessary computations, reduces memory usage, and improves overall performance.
- Reliability: Quality code is less prone to bugs and errors. It undergoes rigorous testing, ensuring that it behaves as intended and produces correct outputs. Reliable code builds trust among users and stakeholders.
Tips for Improving Your Code Quality
Improving the code quality of your Java projects is essential for creating reliable and maintainable software solutions. Here are some valuable tips to help you enhance the code quality in your Java projects:
Writing Clean and Readable Code
Writing clean and readable code is the foundation of high-quality software. Follow these practices to improve code clarity:
- Use meaningful variable and method names: Choose descriptive names that convey the purpose and functionality of each element in your code.
- Break down complex logic: Divide complex tasks into smaller, more manageable functions or methods. This improves code organization and makes it easier to understand and debug.
- Comment and document your code: Add comments to explain the intent and logic behind certain sections of your code. This helps other developers (including yourself) understand and maintain the code in the future.
Implementing Code Reviews and Pair Programming
Code reviews and pair programming are effective techniques for improving code quality through collaboration and feedback:
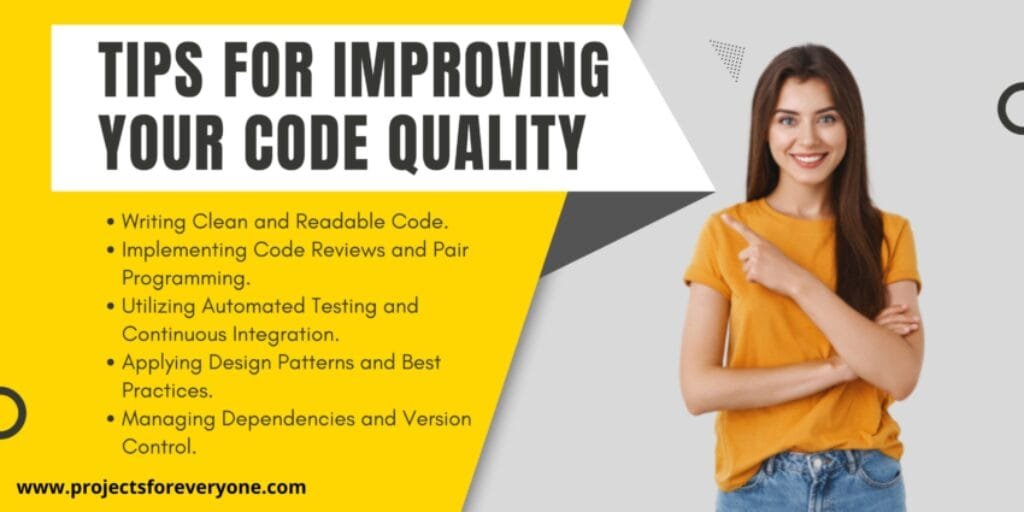
- Code reviews: Regularly conduct code reviews with your team members. This process involves inspecting each other’s code for potential bugs, design flaws, and adherence to coding standards. It promotes knowledge sharing and helps identify areas for improvement.
- Pair programming: Work together with another developer on the same codebase. By sharing ideas, discussing solutions, and reviewing each other’s code in real time, you can catch mistakes early and produce higher-quality code.
Utilizing Automated Testing and Continuous Integration
Automated testing and continuous integration play crucial roles in maintaining code quality throughout the development process:
- Unit testing: Write comprehensive unit tests to verify the correctness of individual components in your Java code. This helps catch bugs and ensures that modifications or additions to the codebase do not introduce regressions.
- Integration testing: Perform integration tests to verify the interaction between different modules or components of your Java project. This helps identify potential issues that arise when multiple parts of the codebase interact.
- Continuous integration (CI): Adopt CI practices by integrating automated testing into your development workflow. CI systems automatically build, test, and validate your codebase whenever changes are committed. This ensures that code quality is continuously monitored and prevents the introduction of faulty code into the project.
Applying Design Patterns and Best Practices
Utilizing design patterns and following established best practices can greatly improve code quality:
- Design patterns: Familiarize yourself with common design patterns in Java, such as Singleton, Observer, and Factory. Applying these patterns appropriately can enhance code structure, maintainability, and reusability.
- Coding standards: Adhere to coding standards and style guidelines specific to Java, such as the Java Code Conventions. Consistent code formatting and indentation improve code readability and make it easier for others to collaborate on the project.
Managing Dependencies and Version Control
Effectively managing dependencies and utilizing version control systems contribute to code quality:
- Dependency management: Use dependency management tools like Maven or Gradle to handle external libraries and frameworks. This ensures consistent versions, prevents conflicts, and simplifies the process of adding or removing dependencies.
- Version control: Employ a version control system such as Git to track changes, collaborate with team members, and maintain a history of your codebase. Version control enables easy reverting of changes, branching for experimentation, and team coordination.
By implementing these tips, you can significantly enhance the code quality of your Java projects and create software that is easier to maintain, more reliable, and less prone to bugs.
Note: If you want to learn about some Tips & Tricks for Java Source Code and Java Projects for Advance Programmers, click on the given link
Tools to Improve Java Code Quality
Improving code quality in Java projects involves leveraging various tools specifically designed to analyze, test, and enhance the codebase. These tools automate processes and provide valuable insights to ensure high-quality code. Here are some essential tools that can help improve code quality in Java projects:
Static Code Analysis Tools
Static code analysis tools analyze source code without executing it, identifying potential issues, and suggesting improvements. Some popular static code analysis tools for Java include:
- SonarQube: SonarQube provides a comprehensive code quality platform that detects bugs, code smells, and vulnerabilities. It offers a wide range of rules and metrics to assess code quality and provides actionable recommendations for improvement.
- FindBugs: FindBugs scans Java bytecode and detects common programming mistakes, potential bugs, and inefficient code patterns. It highlights issues such as null pointer dereferences, infinite loops, and unused variables.
- PMD: PMD analyzes Java source code, identifying potential issues related to code complexity, coding style, and potential bugs. It offers a set of rules to enforce best practices, coding conventions, and maintainability.
Code Formatting and Style Checkers
Consistent code formatting and adherence to coding style guidelines contribute to better code readability and maintainability. Some popular tools in this category include:
- Checkstyle: Checkstyle enforces coding style conventions by analyzing the source code against a predefined set of rules. It checks for consistent indentation, naming conventions, and code formatting.
- Google Java Format: Google Java Format automatically formats Java code according to the Google Java Style Guide. It ensures consistent code formatting across the entire codebase.
Unit Testing Frameworks
Unit testing frameworks facilitate the creation and execution of automated tests, ensuring the correctness and reliability of the code. Some widely used unit testing frameworks for Java include:
- JUnit: JUnit is a popular framework for writing and executing unit tests in Java. It provides a simple and straightforward way to define test cases and assertions, allowing developers to validate the behavior of individual units of code.
- TestNG: TestNG is another powerful testing framework that supports both unit and integration testing. It offers additional features such as data-driven testing, parallel test execution, and configuration flexibility.
Continuous Integration Tools
Continuous integration (CI) tools automate the process of building, testing, and integrating code changes into the main codebase. They ensure that code quality is maintained and issues are detected early. Some commonly used CI tools for Java include:
- Jenkins: Jenkins is a popular open-source CI/CD tool that enables automated building, testing, and deployment of Java projects. It integrates with version control systems and various testing frameworks, allowing you to create custom CI pipelines.
- Travis CI: Travis CI is a cloud-based CI service that automates builds and tests for Java projects. It provides an easy setup process and integrates with popular version control platforms like GitHub.
Code Coverage Tools
Code coverage tools measure the extent to which your code is tested by identifying which parts of the code are executed during testing. They help ensure that the test suite adequately covers the codebase. Some commonly used code coverage tools for Java include:

- JaCoCo: JaCoCo is a popular code coverage library that provides detailed coverage reports for Java applications. It measures line coverage, branch coverage, and cyclomatic complexity, enabling you to identify untested code and improve overall code quality.
- Cobertura: Cobertura is another code coverage tool that generates coverage reports by instrumenting Java bytecode. It helps identify areas of the code that require additional testing and provides insights into the effectiveness of your test suite.
Conclusion
Java projects with source code are widely used in various domains, and ensuring code quality is crucial for their success. In this article, we explored the importance of code quality in Java projects and provided tips for improving it. We discussed the impact of code quality on the maintainability, efficiency, and reliability of software solutions.
Writing clean and readable code, implementing code reviews and pair programming, and utilizing automated testing and continuous integration were among the tips we covered. These practices promote collaboration, catch bugs early, and ensure that the codebase remains robust and maintainable.
For more details visit our site www.projectsforeveryone.com site.
Also, you can contact us at this number:- +918283017879
For more tips and tricks build a project visit our Youtube Channel
Java Project Ideas for Beginners
In case of any doubt, Just try Once and we will not let you down