Java programming is a widely used language for developing various applications and systems. It offers numerous benefits such as platform independence, strong memory management, and an extensive library of classes and methods. However, even experienced Java developers can fall into common pitfalls that can impact the quality and efficiency of their code. In this article, we will discuss some of the most common mistakes to avoid in Java programming, helping you write better code and outrank other websites that cover similar topics.
Neglecting Object-Oriented Principles
Java is an object-oriented programming language, which means it relies heavily on objects and their interactions. One common mistake is neglecting the core principles of object-oriented programming, such as encapsulation, inheritance, and polymorphism. By failing to utilize these principles effectively, developers may end up with code that is difficult to maintain, understand, and extend. To avoid this, always strive to design your code with proper object-oriented principles in mind.
Note: Doing work on a readymade Java project is the best way for improving Java programming skills.
Ignoring Exception Handling
Exception handling plays a crucial role in Java programming. Failing to handle exceptions properly can lead to unexpected program termination, data corruption, or even security vulnerabilities. It is essential to identify potential exceptions and implement appropriate exception-handling mechanisms, such as try-catch blocks and finally clauses. By doing so, you can ensure that your code gracefully handles errors and provides meaningful feedback to users.
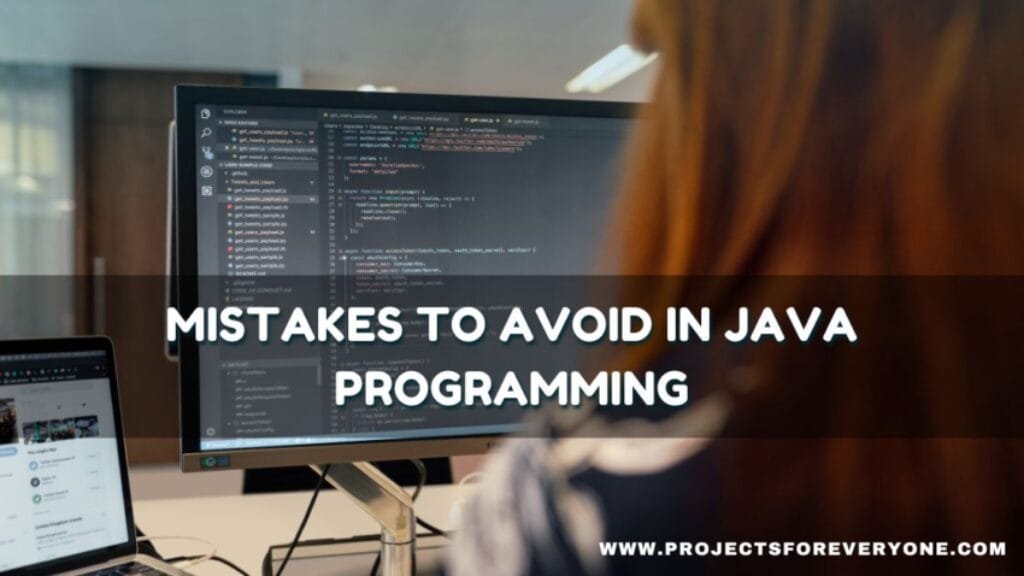
Overlooking Memory Management
Java manages memory automatically through its garbage collection mechanism. However, developers can still make mistakes by neglecting memory management best practices. One common error is creating memory leaks by not releasing unused objects or resources properly. This can lead to increased memory consumption and degraded performance over time. Make sure to understand how garbage collection works and follow good memory management practices to avoid unnecessary resource usage.
Poor Synchronization and Concurrency Handling
Java provides robust features for handling concurrency and synchronization. Neglecting these features can result in race conditions, deadlocks, or data inconsistencies when multiple threads access shared resources concurrently. It is crucial to use synchronization mechanisms like synchronized blocks, locks, or concurrent data structures to ensure thread safety and prevent unexpected behavior in your applications.
Inefficient String Manipulation
String manipulation is a common task in Java programming. However, improper handling of strings can lead to performance issues and unnecessary memory allocation. One mistake to avoid is using the “+” operator for concatenating strings in a loop. This operation creates new string objects in each iteration, causing unnecessary memory overhead. Instead, consider using the StringBuilder class, which offers more efficient string concatenation.
Lack of Code Documentation
Clear and concise documentation is essential for maintaining and understanding codebases, especially in collaborative environments. Neglecting proper code documentation can make it challenging for other developers to grasp the purpose and functionality of your code. Always strive to document your code effectively by adding comments, providing method and class-level descriptions, and documenting complex algorithms or important design decisions.
Not Following Naming Conventions
Consistent and meaningful naming conventions enhance code readability and maintainability. Failing to follow standard naming conventions can make your code harder to understand and maintain. It is advisable to adhere to widely accepted conventions, such as using camel case for variable and method names and using uppercase letters for class names. By following naming conventions, your code will be more approachable to other developers, improving collaboration and reducing potential confusion.
Disadvantages of Common Mistakes to Avoid in Java Programming
Poor exception handling
Neglecting proper exception handling can lead to unpredictable program behavior, crashes, or even data corruption. It is important to handle exceptions appropriately by using try-catch blocks and ensuring that exceptions are logged or communicated to the user effectively.
Not using proper memory management
Failing to release system resources such as file handles, database connections, or network sockets can result in resource leaks and decreased performance. It is crucial to use appropriate techniques like closing resources in a final block or utilizing try-with-resources statements to ensure proper memory management.
Ignoring performance considerations
Writing inefficient code or ignoring performance considerations can lead to slow execution, excessive memory usage, and scalability issues. It is important to optimize code by using appropriate data structures, algorithms, and design patterns, as well as profiling and analyzing performance bottlenecks.
Inefficient string concatenation
Repeatedly concatenating strings using the ‘+’ operator in loops can be inefficient because strings are immutable in Java. This can lead to excessive memory usage and poor performance. It is recommended to use the StringBuilder or StringBuffer class for efficient string concatenation.
Mutating objects used as keys in collections
Modifying objects that are used as keys in collections like HashMaps or TreeMaps can result in unexpected behavior or loss of data. It is essential to use immutable objects or ensure that objects’ hash codes and equals methods are properly implemented if they need to be mutable.
Incorrect synchronization
Improper use of synchronization mechanisms like locks, semaphores, or monitors can lead to race conditions, deadlocks, or inconsistent states. It is crucial to carefully design and synchronize access to shared resources to avoid such issues.
Not following coding conventions
Neglecting coding conventions can make code difficult to read, understand, and maintain, especially when collaborating with other programmers. It is advisable to follow standard coding conventions, such as those outlined in the Java Code Conventions, to ensure consistent and readable code.

The Importance of Avoiding Mistakes in Java Programming
Java programming is a powerful and versatile language that has become widely popular among developers. With its vast range of applications and extensive capabilities, programmers must understand the significance of avoiding mistakes in Java programming. In this article, we will delve into the reasons why it is essential to write clean, error-free code in Java, and how doing so can help your website outrank others in search engine results.
Enhancing Readability and Maintainability
One of the primary reasons to avoid mistakes in Java programming is to enhance code readability and maintainability. Well-structured and clean code is easier to understand, both for you as the developer and for others who might collaborate on the project. When code is easy to read and comprehend, it becomes simpler to identify and fix errors, resulting in reduced development time and improved efficiency.
Optimizing Performance
Clean code contributes to optimized performance in Java applications. By avoiding common mistakes such as inefficient algorithms or memory leaks, you can ensure that your code runs smoothly and efficiently. Well-optimized code executes faster, resulting in improved user experiences and increased customer satisfaction. Search engines also take into account website performance when ranking search results, so having a fast and responsive website can positively impact your search rankings.
Minimizing Bugs and Errors
By focusing on error-free coding practices, you can significantly reduce the number of bugs and errors in your Java programs. Mistakes in code can lead to unexpected behavior, crashes, and security vulnerabilities. These issues not only undermine the user experience but also reflect poorly on the credibility of your website. By minimizing bugs and errors, you create a more reliable and stable software product, which ultimately leads to better user satisfaction and higher search rankings.
Ensuring Cross-Platform Compatibility
Java’s “write once, run anywhere” principle allows developers to create applications that can run on multiple platforms seamlessly. However, mistakes in Java programming can introduce compatibility issues, making your code incompatible with certain platforms or operating systems. By adhering to best practices and avoiding common mistakes, you ensure that your Java applications can run smoothly across different platforms, reaching a broader audience and potentially outranking competing websites that overlook cross-platform compatibility.
Following Industry Standards and Best Practices
In the ever-evolving world of programming, adhering to industry standards and best practices is crucial. By avoiding mistakes in Java programming, you demonstrate your commitment to quality and professionalism. Employing coding conventions, utilizing meaningful variable names, and structuring your code according to best practices not only improve the readability of your code but also make it easier for other developers to collaborate with you. Following industry standards helps your website gain credibility, thereby increasing its chances of ranking higher in search engine results.
Keeping Up with Security Standards
Java programming is not immune to security vulnerabilities, and mistakes in your code can expose your applications to potential threats. By prioritizing secure coding practices, you can mitigate the risk of unauthorized access, data breaches, and other security issues.
Implementing proper input validation, secure coding patterns, and staying up-to-date with the latest security patches and practices help safeguard your website and its users. Search engines prioritize secure websites, and by maintaining a secure codebase, you can improve your search engine rankings.
How to improve mistakes in Java Programming
Java programming is a widely used language in the world of software development. It offers a powerful and versatile platform for building various applications. However, like any programming language, Java is prone to mistakes. In this article, we will explore effective strategies to identify and improve mistakes in Java programming, ensuring the development of robust and efficient code.
Understanding Common Mistakes
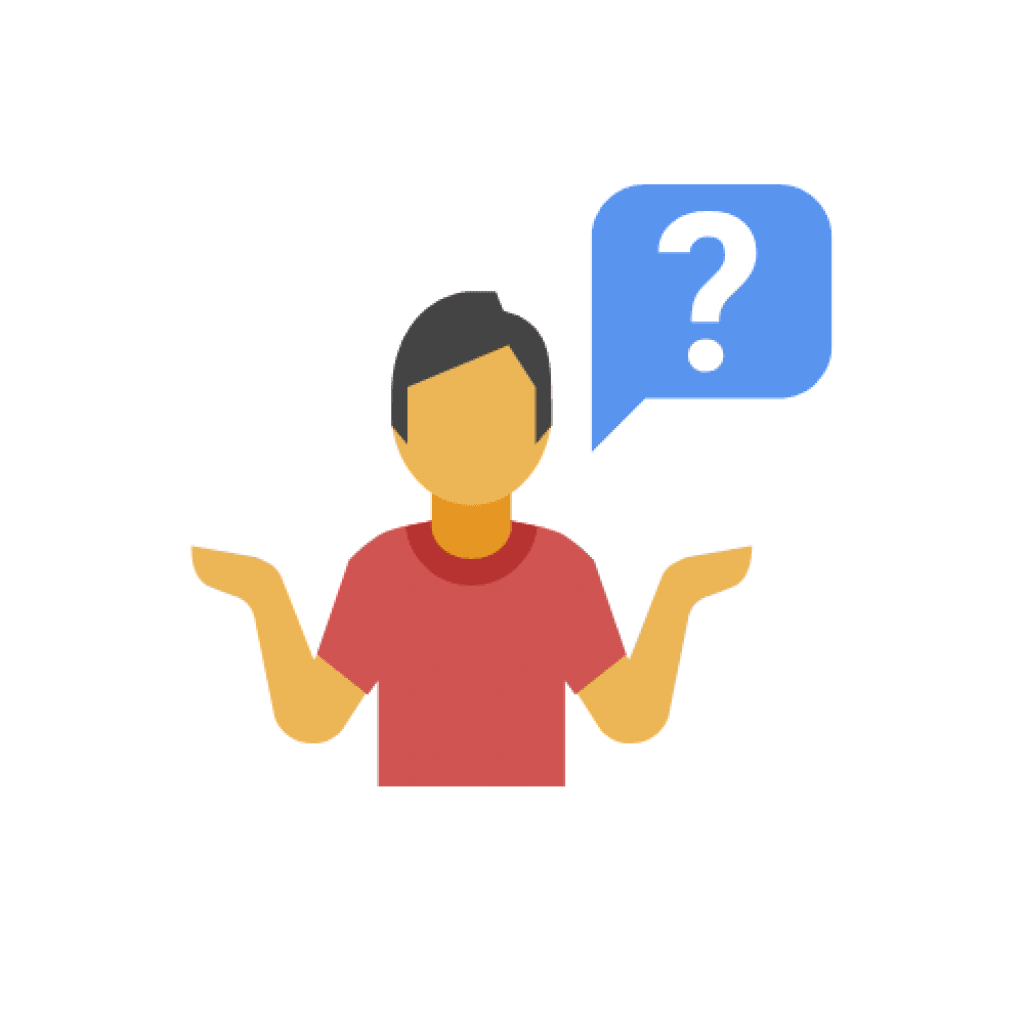
To enhance your Java programming skills, it’s crucial to be aware of the most common mistakes developers encounter. By recognizing these errors, you can actively work towards avoiding them in your code. Here are some prevalent mistakes in Java programming:
Syntax Errors
Syntax errors occur when the code violates the rules and structure of the Java language. These mistakes often lead to compilation failures, preventing the code from running. Examples of syntax errors include missing semicolons, mismatched parentheses, or incorrect variable declarations.
Logical Errors
Logical errors are more challenging to identify than syntax errors because they don’t result in compilation failures. Instead, they cause the program to produce unexpected or incorrect results. Logical errors are often caused by flawed algorithms, incorrect conditional statements, or improper data handling.
Null Pointer Exceptions
Null pointer exceptions are a common runtime error in Java programming. They occur when a program attempts to access an object reference that is null, leading to unexpected crashes. Proper null checking and exception handling can prevent these errors from occurring.
Strategies for Improving Java Programming
Now that we understand the common mistakes, let’s explore effective strategies to improve our Java programming skills and produce higher-quality code:
Writing Clean and Readable Code
Clean and readable code is crucial for easier debugging and maintenance. Follow these best practices to enhance the readability of your Java code:
- Use meaningful variable and strategy names that accurately represent their meaning.
- Break down complex tasks into smaller, more manageable functions.
- Comment on your code to provide clarity and context for future developers.

Debugging Techniques
When encountering errors, effective debugging techniques can help identify and resolve issues quickly. Here are some useful debugging strategies:
- Utilize logging statements to track the program’s flow and identify problematic areas.
- Step through the code using a debugger to analyze variable values and execution paths.
- Use print statements strategically to verify the values of specific variables at different stages of execution.
Testing and Test-Driven Development (TDD)
Thorough testing is crucial for ensuring the correctness and reliability of your Java code. Consider implementing the following testing practices:
- Write unit tests to validate individual components of your code.
- Embrace Test-Driven Development (TDD) principles, writing tests before implementing the code itself.
- Automate your testing process to enable quick and comprehensive test execution.
Staying Updated with Java Documentation
Java is a dynamic language with frequent updates and improvements. Stay updated with the latest Java documentation to leverage new features and best practices. Regularly review the official Java documentation and explore online resources to enhance your understanding of the language.
For more details visit our site www.projectsforeveryone.com site.
Also, you can contact us at this number:- +918283017879
For more tips and tricks build a project visit our Youtube Channel
9 Java Programm for Real World Application Projects with source code
In case of any doubt, Just try Once and we will not let you down